6/30/2025 3:29:03 PM
slxdeveloper.com
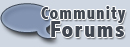 Now Live!
|
|
|
Generically Setting a Form as Read-Only |
|
Description: |
A common request among SalesLogix developers is how to set a form as read-only at runtime. There are far better options than hard-coding each control in a script to disable them. This article will demonstrate how to do this generically using a reusable VBScript class.
|
Category: |
SalesLogix ActiveX Controls
|
Author: |
Ryan Farley
|
Submitted: |
8/2/2005
|
|
|
Stats: |
Article has been read 25661 times
|
Rating:
    - 4.7 out of 5 by 7 users |
|
|
|
fiogf49gjkf0d
A common request among SalesLogix developers is how to set a form as read-only at runtime. There are far better options than hard-coding each control in a script to disable them. This article will demonstrate how to do this generically using a reusable VBScript class.
A common approach used by many to set a form as read only, is to place all controls in a panel. Then when you want the form to be read only, you toggle the Enabled property of the panel. While this is an easy way to get it done, there are still problems with this method. First, the user looses the ability to copy text from the controls since they are inaccessible due to the container being disabled. Second, if your form has buttons to launch other windows, those will be unusable as well.
You might see code every now and then that has a script with all control names hard-coded, disabling each one at a time. I don't like this approach because it is inflexible. As you add more controls to the form, you have to remember to add a corresponding line to the script. It is easy to miss one and cumbersome to keep in sync.
We will look at a building a VBScript class to make the task generic and simple, as well provide a reusable solution for any form.
Note: I originally posted this section of the article on my SalesLogix related weblog at http://saleslogixblog.com/rfarley/archive/2004/07/26/925.aspx
Iterating through controls on a SalesLogix form is is more useful than you might think. It is helpful to be able to generically manipulate all, or a set of controls on a form, to do things such as enable/disable controls at runtime etc without the need to specifically hard-code the names of the controls.
Here's an example. This code iterates through all controls and sets the enabled property to false:
Dim i
On Error Resume Next
For i = 0 To ControlCount - 1
Controls(i).Enabled = False
Next
Easy enough. The SalesLogix form exposed two properties that make this possible. First the ControlCount property will return the number of controls on the form. The Controls collection contains a reference to each of these controls. Note: SalesLogix collections don't work well with For Each loops. Stick with a For loop instead and access collection items by index instead.
You can do anything you want with the control reference in the loop. Finding out which type of control you're working with is easy with the TypeName method. If you pass to it a control reference it will pass back the type of control it is. Here's an example that sets only checkboxes disabled:
Dim i
On Error Resume Next
For i = 0 To ControlCount - 1
If TypeName(Controls(i)) = "CheckBox" Then Controls(i).Enabled = False
Next
Access whatever properties you need once you have the control reference. Often it is useful to use the Tag property to flag controls needed for some specific operation. However, be careful to not access a property while iterating through controls that might not exist on all controls (such as the Text property).
The FormWrapper class will allow you to pass a reference to a form into the class and do operations on that form generically, such as setting all controls on the form as read-only.
One thing to note is that some forms have a ReadOnly property while some do not. What we will do is use the ReadOnly property on the controls that support it, and use the Enabled property on all others. We could just attempt to set a ReadOnly property on any controls we find while iterating the control collection and if it raises an error to then set the Enabled property to false. However, I like to have a little more control over what my script is doing, so I will list each control type and either set ReadOnly or Enabled where appropriate. A special thanks goes to Bob Ledger (aka RJLedger) for taking the time to list the control types and which support ReadOnly.
Class FormWrapper
Private m_form
Public Property Let Form(ByRef val)
m_form = val
End Property
Public Property Let ReadOnly(ByVal val)
SetReadOnly val
End Property
Private Sub SetReadOnly(ByVal ReadOnly)
Dim i
On Error Resume Next
For i = 0 To m_form.ControlCount - 1
Select Case TypeName(m_form.Controls(i))
' Controls that have a ReadOnly property
Case "Edit": m_form.Controls(i).ReadOnly = ReadOnly
Case "Memo": m_form.Controls(i).ReadOnly = ReadOnly
Case "AutoCompleteEdit": m_form.Controls(i).ReadOnly = ReadOnly
Case "DateTimeEdit": m_form.Controls(i).ReadOnly = ReadOnly
Case "LinkEdit": m_form.Controls(i).ReadOnly = ReadOnly
Case "ListView": m_form.Controls(i).ReadOnly = ReadOnly
Case "LookupEdit": m_form.Controls(i).ReadOnly = ReadOnly
Case "NameEdit": m_form.Controls(i).ReadOnly = ReadOnly
Case "PickList": m_form.Controls(i).ReadOnly = ReadOnly
Case "PopupEdit": m_form.Controls(i).ReadOnly = ReadOnly
Case "RichEdit": m_form.Controls(i).ReadOnly = ReadOnly
Case "TreeView": m_form.Controls(i).ReadOnly = ReadOnly
Case "DataGrid": m_form.Controls(i).ReadOnly = ReadOnly
' Controls that must be disabled
' One thing to note, we actually need to negate the ReadOnly parameter passed
' so that the Enabled property is set to False when the form is set to ReadOnly=True
Case "CheckBox": m_form.Controls(i).Enabled = Not ReadOnly
Case "ComboBox": m_form.Controls(i).Enabled = Not ReadOnly
Case "CheckListBox": m_form.Controls(i).Enabled = Not ReadOnly
Case "DateTimePicker": m_form.Controls(i).Enabled = Not ReadOnly
Case "Image": m_form.Controls(i).Enabled = Not ReadOnly
Case "ListBox": m_form.Controls(i).Enabled = Not ReadOnly
Case "MonthCalendar": m_form.Controls(i).Enabled = Not ReadOnly
Case "Panel": m_form.Controls(i).Enabled = Not ReadOnly
Case "ProgressBar": m_form.Controls(i).Enabled = Not ReadOnly
Case "RadioButton": m_form.Controls(i).Enabled = Not ReadOnly
Case "RadioGroup": m_form.Controls(i).Enabled = Not ReadOnly
Case "ScrollBar": m_form.Controls(i).Enabled = Not ReadOnly
Case "Shape": m_form.Controls(i).Enabled = Not ReadOnly
Case "Timer": m_form.Controls(i).Enabled = Not ReadOnly
Case "TrackBar": m_form.Controls(i).Enabled = Not ReadOnly
Case "UpDown": m_form.Controls(i).Enabled = Not ReadOnly
' We are purposely excluding "Button", but if you also want to disable those...
'Case "Button": m_form.Controls(i).Enabled = ReadOnly
End Select
Next
End Sub
End Class
Now to use this class, simply add it as an included script to your form, and add the following code:
Dim frm
Set frm = New FormWrapper
frm.Form = Form 'set this to whatever your form's "name" property is
frm.ReadOnly = True
'Or if you want to make the form editable
'frm.ReadOnly = False
At times some tasks in SalesLogix might seem redundant, but the point is to look for ways you can make things generic and reusable. You'll often find that there are many ways to accomplish something in a SalesLogix script, but taking the time to make it reusable is where the payoff is. Your reusable code library will grow and you'll find yourself being more profitable on projects since you avoid the need to rewrite the same code over and over again.
Until next time, happy coding.
-Ryan
|
|
|
|
Rate This Article
|
you must log-in to rate articles. [login here] 
|
|
|
Please log in to rate article. |
|
|
Comments & Discussion
|
you must log-in to add comments. [login here]
|
|
|
- subject is missing.
- comment text is missing.
|
|
| Re: Generically Setting a Form as Read-Only Posted: 8/3/2005 7:04:37 AM | fiogf49gjkf0d Great info Ryan! This article is not only useful for handling readonly and enable/disable issues but it also is a good example of using a class. | |
|
| Re: Generically Setting a Form as Read-Only Posted: 8/3/2005 10:28:05 AM | fiogf49gjkf0d Thanks RJL. Having it all in a class yeilds other unseen benefits since you can add other form manipulation stuff to the class and now you have this nicely wrapped class that extends the form to add all this extra functionality.
Also, I wanted to point out, that because you pass in a reference to the form, instead of just having this be a function in the form's script itself, that you are able to pass in a reference to other forms also - not just the current form you are on.
So you are able to do something like this:
Dim frm Set frm = New FormWrapper frm.Form = Application.Forms("System:SomeOtherForm") frm.ReadOnly = True
And set other forms as read only as well. Makes it even more flexible.
-Ryan | |
|
| Re: Generically Setting a Form as Read-Only Posted: 8/3/2005 1:32:09 PM | fiogf49gjkf0d Adding my thanks to what RJL already wrote - great article. Tied to what you have previously shown with the history class I have really appreciated the power and flexibility that classes offer the SalesLogix developer - and your clear examples and documents make the learning curve manageable. Thanks again. | |
|
| Re: Generically Setting a Form as Read-Only Posted: 9/27/2005 2:30:46 AM | fiogf49gjkf0d Just a quick question on your example of using the class. Shouldn't there be a Set frm = Nothing at the end? If this is not necessary do you mind taking a moment to explain why you wouldn't need to derefrence a class?
Thanks again for all your great posts and examples.
Ted | |
|
| |
| Re: Generically Setting a Form as Read-Only Posted: 10/18/2007 3:03:10 PM | fiogf49gjkf0d I was able to get this to work on a couple of add/edit forms and it is really slick!!! I was wondering how I could use this functionality with the tab forms. I cannot seem to get it to work on an account tab form. Is this possible to do and if so, could you let me know if or what I need to do differently? Thanks... | |
|
| Re: Generically Setting a Form as Read-Only Posted: 10/18/2007 3:35:26 PM | fiogf49gjkf0d Hi Sarah,
Yes, it does work on account tabs (or any tab form) as well. I'll probably need a bit more info on how you're using it (ie; where is the code being run from, on the account tab or from some other location, etc). Maybe start a forum thread on the topic, I'd be glad to help.
-Ryan | |
|
| Re: Generically Setting a Form as Read-Only Posted: 5/6/2009 10:41:17 AM | fiogf49gjkf0d I want that opportunity as read-only.I have opened the OPPORTUNITY DETAIL in architect.I create the FORMWRAPPER CLASS and i add the istruction for use class but in saleslogix client i can modify the opportunity | |
|
| Re: Generically Setting a Form as Read-Only Posted: 9/23/2009 11:30:32 AM | fiogf49gjkf0d On an Opportunity Tab, SLX is completely ignoring an edit control set to ReadOnly = T.
Only if I txtSalesPotential.READONLY = TRUE during the AXFormchange will it actually prevent me from editing the control (and changing this DATABOUND value).
I thought it might be the script from the Opportunity Detail View (using the above coding example).....even when I changed the name of the control it still is readonly = false....maybe it's a leak in my script and the controls on more than one form are being set???
Is there a form level property that has to be set? frmName.AllowReadOnly = TRUE
harkens back to the Legacy stuff......
This is in 7.22 | |
|
|
|
|
|
Visit the slxdeveloper.com Community Forums!
Not finding the information you need here? Try the forums! Get help from others in the community, share your expertise, get what you need from the slxdeveloper.com community. Go to the forums...
|
|
|
|
|
|