7/9/2025 1:33:00 AM
slxdeveloper.com
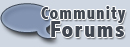 Now Live!
|
|
|
Connecting with the RWPassword From External Applications |
|
Description: |
This article focuses on how you can programmatically determine, decrypt, and use the SalesLogix RWPassword to gain Read/Write access to SalesLogix data from external applications using C# and VB.NET.
|
Category: |
SalesLogix OLE DB Provider
|
Author: |
Ryan Farley
|
Submitted: |
1/14/2003
|
|
|
Stats: |
Article has been read 24319 times
|
Rating:
    - 5.0 out of 5 by 9 users |
|
|
|
fiogf49gjkf0d
This article focuses on how you can programmatically determine, decrypt, and use the SalesLogix RWPassword to gain Read/Write access to SalesLogix data from external applications using C# and VB.NET.
SalesLogix v6 connections defined in the Connection Manager contain an RWPassword to be able to limit Read/Write access to SalesLogix data from outside applications. To use the RWPassord, you simply supply it in the connection string when making your ADO.NET or ADO connection (See Understanding the SalesLogix OLE DB Connection String for more information). If you do not supply this RWPassword, then your connection will be read-only. In an attempt to not cripple the type of external addons that can be developed by SalesLogix Business Partners, SalesLogix provides two options for getting around this security feature from external applications.
The first method for bypassing the need to supply a RWPassword is to set the RWPassword for the connection in the Connection Manager to a blank password. If the RWPassword is blank, it is then no longer required. You don't even need the parameter in the connection string and you'll still gain read/write access to the SalesLogix database. However, you can't really make a generic application that relies on this. Many companies may not like the idea of removing the RWPassword, and you cannot trust that the RWPassword will always be the case (The future version 6.1 of SalesLogix may change this as a new Read-Access password will be introduced as well). The seconds method it to programmatically determine and decrypt the RWPassword.
SalesLogix v6 introduces new SalesLogix-specific procedures that can be executed in the SalesLogix Provider. One of these is the slx_RWPass() procedure. This procedure will return to you the SalesLogix RWPassword. See the following code for an example of it's usage (in both C# and VB.NET):
// ----- C# -----
OleDbConnection conn = new OleDbConnection("Provider=SLXNetwork.1;"
+ "Data Source=SALESLOGIX_EVAL2;"
+ "User ID=Admin;Password=\"\";"
+ "Extended Properties=\"SLX Server=MORPHEUS;"
+ "ADDRESS=localhost;Type=ODBC;PORT=1706\"");
try
{
conn.Open();
OleDbCommand cmd = new OleDbCommand("slx_RWPass()", conn);
MessageBox.Show("RWPassword is: " + cmd.ExecuteScalar().ToString());
}
catch (Exception ex)
{
MessageBox.Show(ex.Message, ex.GetType().ToString());
}
finally
{
if (conn.State == ConnectionState.Open) conn.Close();
conn = null;
}
' ----- VB.NET -----
Dim conn As New OleDbConnection("Provider=SLXNetwork.1;Data Source=SALESLOGIX_EVAL2;" & _
"User ID=Admin;Password=;Extended Properties=SLX Server=MORPHEUS;" & _
"ADDRESS=localhost;Type=ODBC;PORT=1706")
Try
conn.Open()
Dim cmd As New OleDbCommand("slx_RWPass()", conn)
MessageBox.Show("RWPassword is: " & cmd.ExecuteScalar().ToString())
Catch ex As Exception
MessageBox.Show(ex.Message, ex.GetType().ToString())
Finally
If conn.State = ConnectionState.Open Then conn.Close()
conn = Nothing
End Try
If you ran the code, you'll see that although your RWPassword may be set as something like "MonkeyBrains" that the value returned looked more like "7A9CCB7AD60C4CF562ED". This is because the RWPassword has been encrypted. The SalesLogix Developer Reference makes no mention of the fact that this password is returned encrypted, but it also does not mention how to decrypt it, which we will look at now.
When you install SalesLogix, an ambiguously named COM DLL is installed in the SalesLogix directory named SLXRWEL.DLL. This stands for SalesLogix Read/Write Encryption Library and it is used for just that. This COM DLL exposes one public class named SLXRWEOBJ. This class has two public methods, Add - used to add encryption to a RWPassword, and Remove - used to remove the encryption from the RWPassword.
Since we're using .NET for our code examples, you'll need to add a reference to this library by either right-clicking the "Referenced" node of the Solution Explorer, or go to the "Project" menu and select "Add Reference". When the Add Reference dialog appears, select the COM tab and browse to select the SLXRWEL library and add it to the project (see below).
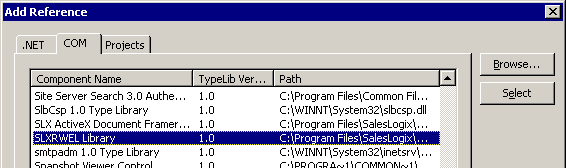
One thing to note here. When adding a reference to a COM object using a.NET language, the .NET IDE will create an Interop library for us. You'll see a new DLL along with your compiled project named Interop.SLXRWEL.dll. Be sure you distribute that DLL along with your application. Now that we've added the reference, we can add it to our code to decrypt the RWPassword. Let's take a look.
// ----- C# -----
OleDbCommand cmd = new OleDbCommand("slx_RWPass()", conn);
string rwpass = cmd.ExecuteScalar().ToString();
SLXRWEL.SLXRWEOBJ rw = new SLXRWEL.SLXRWEOBJClass();
rwpass = rw.Remove(rwpass);
MessageBox.Show("RWPassword is: " + rwpass);
' ----- VB.NET -----
Dim cmd As New OleDbCommand("slx_RWPass()", conn)
Dim rwpass = cmd.ExecuteScalar().ToString()
Dim rw As New SLXRWEL.SLXRWEOBJ()
rwpass = rw.Remove(rwpass)
MessageBox.Show("RWPassword is: " & rwpass)
That was easy enough. You'll notice that this time, the value displayed in your MessageBox is the correct, unencrypted RWPassword. But now, what do you do with it?
Now that you have successfully retrieved and decrypted the RWPassword, you need to use it to re-connect to the database. In our sample above, we made a connection to the database to be able to execute the slx_RWPass() procedure. This connection we made to do this was read-only since we did not have the RWPassword to supply in the connection string. What we need to do now that we have the RWPassword, is disconnect our current connection and then re-connect, this time supplying the RWPassword in our connection string. Let's look at the whole thing all together now:
// ----- C# -----
OleDbConnection conn = new OleDbConnection("Provider=SLXNetwork.1;"
+ "Data Source=SALESLOGIX_EVAL2;"
+ "User ID=Admin;Password=\"\";"
+ "Extended Properties=\"SLX Server=MORPHEUS;"
+ "ADDRESS=localhost;Type=ODBC;PORT=1706\"");
try
{
// open read-only connection
conn.Open();
OleDbCommand cmd = new OleDbCommand("slx_RWPass()", conn);
// retrieve encrypted RWPassword
string rwpass = cmd.ExecuteScalar().ToString();
SLXRWEL.SLXRWEOBJ rw = new SLXRWEL.SLXRWEOBJClass();
// descrypt the RWPassword
rwpass = rw.Remove(rwpass);
// Now close the connection and re-open it using the RWPassword
conn.Close();
conn = new OleDbConnection("Provider=SLXNetwork.1;"
+ "Data Source=SALESLOGIX_EVAL2;"
+ "User ID=Admin;Password=\"\";"
+ "Extended Properties=\"SLX Server=MORPHEUS;"
+ "ADDRESS=localhost;Type=ODBC;PORT=1706;"
+ "RWPass=" + rwpass + ";\"");
conn.Open();
MessageBox.Show("Our connection is now a read/write connection!");
}
catch (Exception ex)
{
MessageBox.Show(ex.Message, ex.GetType().ToString());
}
finally
{
if (conn.State == ConnectionState.Open) conn.Close();
conn = null;
}
' ----- VB.NET -----
Dim conn As New OleDbConnection("Provider=SLXNetwork.1;Data Source=SALESLOGIX_EVAL2;" & _
"User ID=Admin;Password=;Extended Properties=SLX Server=MORPHEUS;" & _
"ADDRESS=localhost;Type=ODBC;PORT=1706")
Try
' open read-only connection
conn.Open()
Dim cmd As New OleDbCommand("slx_RWPass()", conn)
' retrieve encrypted RWPassword
Dim rwpass = cmd.ExecuteScalar().ToString()
Dim rw As New SLXRWEL.SLXRWEOBJ()
' descrypt the RWPassword
rwpass = rw.Remove(rwpass)
' Now close the connection and re-open it using the RWPassword
conn.Close()
conn = New OleDbConnection("Provider=SLXNetwork.1;Data Source=SALESLOGIX_EVAL2;" & _
"User ID=Admin;Password=;Extended Properties=SLX Server=MORPHEUS;" & _
"ADDRESS=localhost;Type=ODBC;PORT=1706;RWPass=" & rwpass)
conn.Open()
MessageBox.Show("Our connection is now a read/write connection!")
Catch ex As Exception
MessageBox.Show(ex.Message, ex.GetType().ToString())
Finally
If conn.State = ConnectionState.Open Then conn.Close()
conn = Nothing
End Try
There you go. You now have a Read/Write connection to the database for you do use as needed - without ever needing to ask someone what their RWPassword is!
Yes it is. The purpose of the RWPassword is not to have some iron-clad security, preventing everyone from gaining Read/Write access to the SalesLogix data. Remember, you still need to supply a valid SalesLogix user name and password to connect at all. If someone has a valid user name & password, they can just log into the Sales Client, so why not let them also view & update the data from your cool external addon for SalesLogix too? The purpose for the SalesLogix RWPassword is to prevent Joe User, who might know something such as MS Access, from getting in via a connection from Access and mucking up the data. Besides, if you take the Business Partner version 6 recertification exam, this question is on the test (although it has not been documented anywhere that I've seen yet).
Using the techniques in this article, you can start building cool external applications and addons for SalesLogix - with Read/Write access to the data. So let's get coding! Keep in mind that the information described here may change with version 6.1 of SalesLogix when the new Read-Access password is introduced. I'll update this article as soon as I get the information to reflect any changes.
Until next time, happy coding.
-Ryan
|
|
|
|
Rate This Article
|
you must log-in to rate articles. [login here] 
|
|
|
Please log in to rate article. |
|
|
Comments & Discussion
|
you must log-in to add comments. [login here]
|
|
|
- subject is missing.
- comment text is missing.
|
|
| Good Article Ryan! Posted: 1/15/2003 7:46:46 AM | fiogf49gjkf0d Thanks for the data. It is a bit disconcerting that SLX would put this on the re-cert exam(which I am yet to take) but I am glad you caught it.
Thanks again!, Richard Weck Harris Technology | |
|
| Good to know Posted: 1/15/2003 8:17:37 AM | fiogf49gjkf0d Hey Ryan, good article! Wish I knew this yesterday during the exam! :) | |
|
| Thanks... Posted: 1/15/2003 9:04:09 AM | fiogf49gjkf0d Thanks guys. It was a little sneaky to put it on the re-cert exam since it hasen't been documented anywhere (at least no where that I've come accross yet - and I've read about all the docs released in the SDK). I actually wrote this article a while ago but was holding off on putting it on the site. I was not sure if SLX wanted the information known by the masses - especially when it did not appear in the docs. But, hey, if it's on the test you're going to have to learn about it somewhere ;-)
-Ryan | |
|
| Thank you Ryan! Posted: 1/16/2003 5:10:18 PM | fiogf49gjkf0d Great article! As you say this is not documented anywhere so I had to struggle a while to find out why the connection was read only and where to change that. I think this site is going to be my first place to look for answers about SLX programming from now on. | |
|
| Syncing changes to remotes Posted: 4/21/2003 1:56:00 PM | fiogf49gjkf0d With all this coding going on for External apps, do these changes or updates create TEFS for the remotes?
| |
|
| Re: Syncing changes to remotes... Posted: 4/26/2003 2:29:17 PM | fiogf49gjkf0d With all this coding going on for External apps, do these changes or updates create TEFS for the remotes?
Absolutely! In the code in this article we are using the SalesLogix OLEDB Provider, anything we write to the database via the provider will synchronize, just as if it was done through the sales client. Essentially, the sales client writes to the database in the same way, just be performing inserts/updates/etc via the provider. The provider takes care of the creation of sync logs.
In versions prior to v6, you can still write sync aware to the database, but since the provider does not exist for these older versions, you would need to write all data to the database via the SlgxApi.DLL instead of ADO or ADO.NET.
Don't be afraid to do things outside of SalesLogix - it is a great way to break the barriers of the sales client and develop some really great addons for SalesLogix.
-Ryan
| |
|
| Re: Connecting with the RWPassword From External Applications Posted: 3/11/2004 8:22:26 AM | fiogf49gjkf0d If I were to insert data from a web form, does the provider need to be installed on the webserver, or can I call the instance installed on my SalesLogix server? The LocalHost bit has me confused.
-Micah | |
|
| Re: Connecting with the RWPassword From External Applications Posted: 3/11/2004 8:45:31 AM | fiogf49gjkf0d What you need to understand is there are two different services. One is the OLEDB Provider Engine which runs in every workstation and the other one is the SLX Server. The Provider in your local PC talks to the service located in the SLX Server. In your case you would definitely need to install the OLEDB Provider in the web server. You can install only that if you don't want any client, look for the MSI file in the root directory on your installation CD.
That said, if you change the localhost entry to another ip/name you can actually use the OLEDB Provider in other PC. It's slow and causes problems but it's feasible, I wonder why they left that open... | |
|
| Re: Connecting with the RWPassword From External Applications Posted: 3/11/2004 8:04:59 PM | fiogf49gjkf0d Carlos & Micah,
The localhost thing is actually from the original beta architecture of the provider. This was changed in the final release of v6 and is not meant to be anything other than 'localhost'. Even changing it to something else - you would still need the provider installed locally anyway so it knows what 'SlxNetwork.1' means.
Micah, take a look at this article discussing the SLX connection string for more info:
http://www.slxdeveloper.com/page.aspx?action=viewarticle&articleid=7
Carlos is correct, you would need the provider installed on the web server in order to use the SLX provider and have things synchronize. | |
|
| Re: Connecting with the RWPassword From External Applications Posted: 8/19/2004 1:02:30 PM | fiogf49gjkf0d Hi I am trying to connect to SLx via the provider from a server. There is a firewall between the two servers. Ports 1706, 1707 and 1433 are open. The problem is I keep receiving the following error message OpenRDA OLE DB error '80004005'
RDA error: Resource not available - SalesLogix OLEDB Security - Open Connection [DBNETLIB][ConnectionOpen (Connect()).]SQL Server does not exist or access denied
What could be causing this?
| |
|
| Re: Connecting with the RWPassword From External Applications Posted: 8/29/2005 3:38:53 PM | fiogf49gjkf0d Bob ,Are you able to access SQL server from the machine you are trying to connect to ?
If not , try to connect to the sql...Maybe you have to add an alias or change you registry to do that..
registryWrite "HKLM\SOFTWARE\Microsoft\MSSQLServer\Client\SuperSocketNetLib\Tcp\DefaultPort",3341,"REG_DWORD"
OR
registryWrite "HKLM\SOFTWARE\Microsoft\MSSQLServer\MSSQLServer\SuperSocketNetLib\Tcp\TcpPort",3341,"REG_SZ"
FYI i was using 3341 port for my sql..pls change it to whatever you have
| |
|
| Re: Connecting with the RWPassword From External Applications Posted: 10/31/2007 10:34:00 AM | fiogf49gjkf0d Hi !! i'm a new user of SLX and I'm french so i'm sorry for my english.
I have a question about this fonction, I want modify the password by an application developped in Visual Basic 6 and i want to know if is possible to do this. And if Yes can you help me, please. Thank you | |
|
|
|
|
|
Visit the slxdeveloper.com Community Forums!
Not finding the information you need here? Try the forums! Get help from others in the community, share your expertise, get what you need from the slxdeveloper.com community. Go to the forums...
|
|
|
|
|
|