7/26/2024 9:58:49 PM
slxdeveloper.com
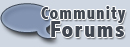 Now Live!
|
|
|
How to generically invoke the edit form for a SalesLogix datagrid |
|
Description: |
Many people miss the older (pre version 6) behavior of SalesLogix grid's where the user could double-click on a row to invoke the grid's edit form. The new datagrids to not behave this way because of the new support for inline editing and activating the clicked cell instead of doing something like invoking the edit form. However this is easy enough to change and handle with a generic script that can be included when ever you need this functionality.
|
Category: |
Architect How To Articles
|
Author: |
Ryan Farley
|
Submitted: |
3/23/2004
|
|
|
Stats: |
Article has been read 31506 times
|
Rating:
    - 5.0 out of 5 by 12 users |
|
|
|
fiogf49gjkf0d
Many people miss the older (pre version 6) behavior of SalesLogix grid's where the user could double-click on a row to invoke the grid's edit form. The new datagrids to not behave this way because of the new support for inline editing and activating the clicked cell instead of doing something like invoking the edit form. However this is easy enough to change and handle with a generic script that can be included when ever you need this functionality.
The ShowViewForRecord method in SalesLogix provides a way to invoke a data-bound form and provide the context of which row to display data for. This method is required when invoking a data form, or the edit form for a datagrid because it allows up to specify which record to display in the form, such as the record represented by the row in the grid that was double-clicked. ShowViewForRecord can be found in the Application.BasicFunctions object exposed in VBScript plugins and is defined as follows:
ShowViewForRecord(TableName As String, FormName As String, RecordID As String) As ModalResult
Let's take a quick look at each of the parameters:
- TableName
This is the base table for the data form you wish to invoke. When you create a data form in the Architect you select the table that the data on the form is based on. This is the value you pass for this parameter.
- FormName
The full name of the data form you wish to invoke. The full name needs to include both the family name and the form name, such as "System:My Data Form".
- RecordID
This is the record you wish to display the data for. If this parameter is blank, then the data form opens in "Add" mode. Otherwise it opens in "Edit" mode for the record ID that was passed for the parameter.
The ShowViewForRecord returns a ModalResult value. A ModalResult can be one of the following:
- mrNone
- mrOk
- mrCancel
- mrAbort
- mrRetry
- mrIgnore
- mrYes
- mrNo
- mrAll
- mrNoToAll
- mrYesToAll
The ModalResult that is returned from ShowViewForRecord tells you what action the user did to close the form. For example, if the user clicked "OK" to save the data then a value of mrOk is returned.
With what you now know about ShowViewForRecord you could easily invoke the edit form for a grid. But why write the same code everytime? Let's look at a few of the properties of the datagrid to see how we can generically invoke the edit form from a script we can include when we need this behavior.
- datagrid.GetCurrentField
The GetCurrentField method on the datagrid will return a field value for the currently selected row in the grid. You can specify a field name or leave it blank to return the default field, which is the keyfield of the data bound to the grid.
- datagrid.KeyField
This property returns the name of the Key or ID field of the data that is bound to the grid. For example, if I had a grid bound to the ACCOUNT table, the value of this property would be "ACCOUNTID". We'll also use this property to give us the table name that the data is based on in the grid. Since SalesLogix key fields are in most cases the TABLENAME + ID this will give us what we need by just trimming off the "ID" at the end of the field name. The only exception to this is a small handful of out of the box tables that use some other name for the table ID.
- datagrid.EditView
This property returns an object that represents the edit form for the grid. All we want is the form's plugin name so we can pass that to ShowViewForRecord. We can use the Name property of this object to return this. So if we use the property like this: datagrid.EditView.Name we will get back a value something like "System:My Form".
Let's put this all together to call ShowViewForRecord without hardcoding any of the parameter values by using these properties:
With DataGrid1
Application.BasicFunctions.ShowViewForRecord Left(.KeyField, Len(.KeyField) - 2), .EditView.Name, .GetCurrentField("")
End With
In that example we used only property values from the grid to call ShowViewForRecord. This will get us closer to using this generically. However, before we move on, let's not forget about that return value. The return value tells us if the user clicked OK - meaning that the user changed & saved the data. In this case, let's refresh the grid to allow the grid to display the modified or new data.
With DataGrid1
If Application.BasicFunctions.ShowViewForRecord(Left(.KeyField, Len(.KeyField) - 2), .EditView.Name, .GetCurrentField("")) = mrOK Then
.Refresh
End If
End With
Now that's pretty cool. We can now put this in a sub that takes the datagrid control as a parameter. This way we don't have to hardcode the 'datagrid1' part in it. Here's the full method we can put into a reusable VBScript plugin:
Sub DataGridEditRow(Grid)
With Grid
If Application.BasicFunctions.ShowViewForRecord(Left(.KeyField, Len(.KeyField) - 2), .EditView.Name, .GetCurrentField("")) = mrOK Then
.Refresh
End If
End With
End Sub
So how do we make this more generic so we can use this all over? Let's not forget that the whole reason we are doing this is so we can use this when a user double-clicks a row in a datagrid. In this case the datagrid's OnDblClick will be raised and a single parameter of Sender will be passed into the event handler. The Sender is a reference to the grid that raised the event. We'll use this reference to pass off to our new DataGridEditRow subroutine. All we have to do is include our VBScript plugin containing the sub and then pass the Sender in the grid's OnDblClick event to the sub.
Sub MyGrid1DblClick(Sender)
DataGridEditRow Sender
End Sub
With the information you now have, you can build a generic script to reuse when ever you need the functionality of invoking a grid's edit view when the user double-click's a row. Reusability is where it is at! You should be looking at generic cases for all the code you write for SalesLogix. Why write the code every time when you have the ability to minimize your development time with your library of reusable scripts? Get the most out of your development and propsper.
Until next time, happy coding.
-Ryan
|
|
|
|
Rate This Article
|
you must log-in to rate articles. [login here] 
|
|
|
Please log in to rate article. |
|
|
Comments & Discussion
|
you must log-in to add comments. [login here]
|
|
|
- subject is missing.
- comment text is missing.
|
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 3/23/2004 7:00:38 PM | fiogf49gjkf0d Excellent article Ryan. I had to work this all out on my own about 6 months ago. You did a great job of explaining it as well as good clear example code.
| |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 3/24/2004 5:46:45 PM | fiogf49gjkf0d I did some testing for the ITtoolbox SalesLogix group and found that if you omit the RecordID you simulate the old DBGridAddValue function.
Try this:
Sub DataGridAddRow(Grid) With Grid If Application.BasicFunctions.ShowViewForRecord(Left(.KeyField, Len(.KeyField) - 2), .EditView.Name, "") = mrOK Then .Refresh End If End With End Sub
I forgot the ModalResult value and usually didn't refresh. The GUI seemed to refresh for me but I may not have noticed it. Thanks again for the tips | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 3/26/2004 3:04:43 PM | fiogf49gjkf0d I have always hard coded my values in this function. I like this 'generic' approach you mention...will put this to use right away!!! Thanks for the tip!!!
| |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 4/15/2004 12:56:16 PM | fiogf49gjkf0d Have to have for the next step where I use views to fead my grid! | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 5/20/2004 2:08:35 PM | fiogf49gjkf0d Fantastic article! In the past, I've used "ShowViewForRecord" to invoke the edit form for a different edit view from the one normally used for a displayed row on a grid. In that case, the displayed record was a linkage record ("many-to-many" relationship) on a Contact tab grid to an Account related table record. But I had to jump through hoops to get an edit view going via a double click on a grid row. Your method for doing this will save me lots of time and effort. I've already put the generic code into an include script and it works just fine. Thanks again. | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 8/17/2004 10:59:03 AM | fiogf49gjkf0d Great Article on Editing a row. The only question if have is how to add using this function. When I leave the RecordID field blank it does not save the information. In other words it does not seem like its binding to the table the way the edit function is. Any assistance on this is greatly appreciated. | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 8/17/2004 11:31:10 AM | fiogf49gjkf0d Sean,
As long as the grid is properly set-up then you can add in the same way mentioned in this article (which is also mention in the comments here by Jeremy) by passing a blank value as the ID to ShowViewForRecord. If the grid is not set up correctly then you'd have problems as you would not be able to grab the right values generically from the grid's properties.
-Ryan | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 8/17/2004 12:34:56 PM | fiogf49gjkf0d What is very odd is that if you right click and choose add the data is added without issue, however the showveiwforrecord function will call the add/edit data form but will not populate the account field with the current account like it does when you right click and choose add. I'm not sure why it would work in one place but not the other. | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 8/17/2004 1:40:46 PM | fiogf49gjkf0d Sean,
It does work exactly the same way both ways for me, whether invoked via right-click or from a call to ShowViewForRecord. Try explicitly passing the values you need to the call to ShowViewForRecord (instead of dynamically getting the properties from the grid) and see if you get the same results. If you do then possibly something is wrong with the Form, if not then something might be wrong with the setup of the grid.
-Ryan | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 8/17/2004 11:31:52 PM | fiogf49gjkf0d In Service Pack 2 using the function I pasted doesn't work. Period. I believe at one time (pre SP2) it worked but now it doesn't.
What it does is kind of weird. It will load the EditView of the grid in question. The form will be blank and appear EXACTLY as it does when you select Add. The funny part is that the data doesn't save at ALL. You fill in the record, click OK and go to look for the record. You won't find any new record. I even went as far as to populate the primary key field programmatically (using OnChange event) and it STILL didn't save the record.
From appearances it looks like it works fine. It shows "Inserting (Form Caption)" instead of "Editing (Form Caption)" but the code behind the call doesn't save the record like it should. Maybe this is a bug? I could have swore it worked before SP2 but I may not have tested it fully. I do know I tested the crap out of it later trying to make it save but I couldn't get it to work.
Ryan if you do have this working I'd be anxious to know which version of SalesLogix you're using. A proof of concept bundle of both the edit and add functions would be cool. I made one up but because I couldn't get the add working correctly I scrapped it and I'm starting to wish I hadn't. | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 8/18/2004 10:49:12 AM | fiogf49gjkf0d Jeremy,
That is strange. I do know for a fact that it *was* working properly pre-SP2. I haven't verified it or anything on a system with SP2 installed yet. I'm out of my office currently doing a DTS class so can't check it now, but I'll do so when I am back in my office to we can get it reported as a bug if needed.
Thanks, -Ryan | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 10/29/2004 4:48:13 PM | fiogf49gjkf0d I am running 6.1 SP2 and I did not have any problems getting this to work. | |
|
| any idea how to trigger a delete row from code for a datagrid Posted: 10/29/2004 4:57:57 PM | fiogf49gjkf0d I know I could call a SQL command to delete the record but I'd like to be able to trigger the delete action on the data grid. instead | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 3/22/2005 1:01:20 PM | fiogf49gjkf0d How about when the client asks for a "DELETE" button to be equivilant to the right-click Delete option? Is there a way to tell the datagrid to delete the currently selected row (after prompting the user for confirmation)? The other option would be to query the datagrid for the currentID and then execute a Delete statement. The latter is much less desireable... | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 3/22/2005 1:18:00 PM | fiogf49gjkf0d Jonathan,
There's no built in way. You just read the ID for the selected row (or rows) and execute the necessary delete statement(s). You can still make it generic, reading the table name, ID field, etc from the grid control.
-Ryan | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 3/22/2005 1:55:05 PM | fiogf49gjkf0d Thanks Ryan. I was suspecting that, but in the back of my mind was hoping to be wrong. At least I know where I stand. The API does leave much to be desired! | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 3/31/2005 1:26:05 PM | fiogf49gjkf0d Well, I have been using this code of Ryan's for a long time. But a customer pointed out to me today something that I guess is obvious, but only an idiot would do it. If the user double-clicks on the grid and no rows are there the user gets an error. | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 3/31/2005 1:31:29 PM | fiogf49gjkf0d Hi Scott,
Yeah, I came accross that same thing. I added a check to make sure that .GetCurrentField <> "" before attempting the invoke which made it an easy fix. The best part is that if you're using this in a reusable include script then you just need to fix the one place and it effects every grid that uses it.
-Ryan | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 7/28/2005 5:21:55 PM | fiogf49gjkf0d hi this is an emergency if any one can help me y do all those step and it work fine its open diferent edit windows for diferent values in the data grid with some if clauses the first time work perfect with this code Sub EntityListDblClick(Sender) select case entitylist.GetCurrentField("type") case "OFFSHORE COMP." entitylist.EditView.name="Personal:Offshore Edit" entitylist.KeyField = "OFFSHOREID" With entitylist If Application.BasicFunctions.ShowViewForRecord(Left(.KeyField, Len(.KeyField) - 2), .EditView.Name, .GetCurrentField("OFFSHOREID")) = mrOK Then .Refresh end if End With case "TRUST COMP." entitylist.EditView.name="Personal:Trust Info Edit" entitylist.KeyField = "TRUST_INFOID" With entitylist If Application.BasicFunctions.ShowViewForRecord(Left(.KeyField, Len(.KeyField) - 2), .EditView.Name, .GetCurrentField("TRUST_INFOID")) = mrOK Then .Refresh end if End With end select End Sub
but the second time i choose a diferent row with different .KeyField and entitylist.GetCurrentField(xxx) return the same value that the first time and stay with that value until you close saleslogix any subjestions?
thanks jose matheus
| |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 7/29/2005 11:32:12 AM | fiogf49gjkf0d If I understand the problem, when you double click on the grid it will store the GetCurrentField("") value. When you change to another record and double click again, that same GetCurrentField from the first click shows up. If this is the case, comment out as much as possible until you return the correct values when you double click. Once that's been done, then add your extra code and test it from there. If you can't get it to work with the entire code, then you know that it is pulling the right value but something you're doing isn't getting it to update.
For the record, GetCurrentField("") (with nothing in the quotes) always returns the .KeyField value or at least it should. That may only be with datagrids that were done at design time and not runtime though, so I may be wrong on that. This means you could probably change your code so that you're making the exact same call twice like this:
Sub EntityListDblClick(Sender) dim stringKeyFieldValue, stringType stringType = Sender.GetCurrentField("TYPE") select case stringType case "OFFSHORE COMP." Sender.EditView.Name="Personal:Offshore Edit" Sender.KeyField = "OFFSHOREID" case "TRUST COMP." Sender.EditView.Name="Personal:Trust Info Edit" Sender.KeyField = "TRUST_INFOID" end select With Sender .Refresh If Application.BasicFunctions.ShowViewForRecord(Left(.KeyField, Len(.KeyField) - 2), .EditView.Name, .GetCurrentField("")) = mrOK Then .Refresh end if End With End Sub
This eliminates the need to call ShowViewForRecord twice, also refreshes the grid after KeyField and EditView.Name is set just in case it's needed for the Edit to fire correctly. I changed entitylist to Sender, since that is always the datagrid who's DoubleClick event just fired. This means you can copy/paste this code into another script without modifying much at all.
Finally, I added the stringType variable to catch the GetCurrentField("type") call. This is most likely the cause of your problem as I found that sometimes if you didn't use a variable it would tend to stick with the first value called. It's been a while since that was a problem for me, so I could be wrong. Hopefully this is a good starting point or maybe it fixed the problem entirely. | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 9/6/2005 10:27:26 AM | fiogf49gjkf0d Ryan, I found a situations where I sometimes needed to have my datatgrid RO and sometime RW. So in order to be able to use the "generic" approach under these conditions: Sub DataGridEditRow(Grid) With Grid If ((.EditOptions.ShowEdit = False) or (.EditOptions.ShowAdd = False)) Then Exit Sub Else If Application.BasicFunctions.ShowViewForRecord(Left(.KeyField, Len(.KeyField) - 2), .EditView.Name, .GetCurrentField("")) = mrOK Then .Refresh End If End If End With End Sub -- RJLedger
| |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 9/6/2005 11:08:51 AM | fiogf49gjkf0d That's a great idea RJL. I'll have to add that into my toolbox version of this script.
-Ryan | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 11/15/2005 3:04:30 PM | fiogf49gjkf0d Has anyone found any SalesLogix documentation on the properties and methods available for the various objects? You can see the options in the object browser simply enough, but it'd sure be nice to see what they're all supposed to do.
This article was great. In fact, after looking at the complete garbage contained in many of the SalesLogix forms it makes me wonder whether Best should just fire their internal development staff and hire their business partners to create future versions.
But that aside, I'm trying to get the grid to fire the edit view and do an add for me. Why would I do a silly thing like that you ask? Because we're moving from 5.2 and trying not to recode every bloody customized form all at once. The ActiveX grid control is quite capable of working properly with a legacy form set to it's edit view, but the "ShowViewForRecord" call will only accept new forms. I've found that using the grdMyGrid.PopupMenu.Items(0).Click method will work like a charm, but ONLY if the user right-clicked on the grid at some point after the form was loaded. Otherwise you get an "array index out of bounds" error because apprently the Items collection is empty until the user right-clicks for the first time. Without any doc I can't figure out how to get the grid to initialize its PopupMenu.
Yes, it's nice to be back to full-blown VBscript, but you have to admit there was a certain appeal to a simple function call:
dbGridAddItem "grdMyGrid"
Sigh. Those were the days, eh? | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 11/15/2005 3:41:54 PM | fiogf49gjkf0d OK, Ok. Now that I've been publicly exposed as a dork, allow me to mitigate the damage by being the first one to point that fact out. Yes, ShowViewForRecord does in fact work with legacy views as well as new ones. I could've sworn I read in the doc that it was only for new forms, but I've looked again and it doesn't say that. The only consolation is that I'm not at the bottom of the geek pile - SalesLogix SDK support didn't suggest ShowViewForRecord as a solution, nor could they tell me why the PopupMenu wasn't loading... | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 8/24/2006 12:34:41 PM | fiogf49gjkf0d Ryan... i love you | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 8/24/2006 12:37:54 PM | fiogf49gjkf0d Josh,
Haha. :-)
-Ryan | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 12/18/2006 7:15:36 PM | fiogf49gjkf0d Here's what I've been using since your article was first published....
Sub DataGridEditRow(Grid) dim tname With Grid If ((.EditOptions.ShowEdit = False) or (.EditOptions.ShowAdd = False)) Then Exit Sub Else tname = Left(.KeyField, Len(.KeyField) - 2) SELECT CASE tname CASE "OPPPRODUCT" tname = "OPPORTUNITY_PRODUCT" CASE "LITREQ" tname = "LITREQUEST" END SELECT If Application.BasicFunctions.ShowViewForRecord(tname, .EditView.Name, .GetCurrentField("")) = mrOK Then .Refresh End If End With End Sub
you can easily add more ODDBALL tables, as needed.
One 'problem' that needs to be pointed out.....this routine does NOT invoke an OnEditedRow/OnAddedRow event on a datagrid. The normal LMB on the grid choose Edit does invoke the OnEditedRow....but this generic ShowViewForRecord call doesn't.
You'll need to explicitly call your cleanup routine after you use this datagrid edit call..... (now that's why my Sum of the Opportunity_Product script didn't run!!!).
RJ Samp | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 2/24/2011 9:36:03 AM | fiogf49gjkf0d I've found a rather big hole in this which I'm hoping someone can help me with.
I'm trying to invoke the edit form tied to a DBGrid in "Add Mode" rather than edit mode, and have done as you suggested because as far as I can see you can't get the DB Grid to invoke it's own edit form like you used to in the old days.
Application.BasicFunctions.ShowViewForRecord "TABLE2", "TABLE2EDITVIEW", ""
This does indeed pull up the correct form in "Add" mode.
However, the DBGrid is 'bound' to another table (TABLE1).
If I manually click on the DBGrid and select "Add", the edit view opens up perfectly in 'Add Mode' and the link back to TABLE1 is already in place (as such, any other edit boxes I have dropped on the form that reference back to TABLE1 prepopulate nicely).
The pain is, if I invoke this form using the ShowViewForRecord code, the secondary key / binding linking it back to TABLE1 is NOT prepopulated. As such, any edit boxes I have dropped on the form that reference back to TABLE1 are blank!
Is there an easy way around this without having to throw global variables around.
(PS. v7.2.2.1871)
Thanks! :-) | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 2/24/2011 9:44:45 AM | fiogf49gjkf0d Guy,
The ShowViewForRecord method doesn't have any ties to a grid at all, so it shouldn't/won't matter what table the grid is bound to since ShowViewForRecord doesn't have anything to do with the grid, or even know about it. It all depends on what the base table of the data bound form is and what table value you pass to ShowViewForRecord.
Does that make sense? | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 2/24/2011 10:06:02 AM | fiogf49gjkf0d Indeed it does, thank you, but that's precisely my point.
I'm trying to simulate exactly the same thing that a DBGrid does when you click on the "Add" item in the menu, ShowViewForRecord does not seem to achieve this. When clicking "Add" on the DBGrid it manages to pass the bindings over to the form, so that edit boxes linked to other tables within the form can populate themselves even when it's a new record you're adding. The ShowViewForRecord function does not provide an argument for establishing the foreign key.
I have two data grids..
GRID1 (gets info from TABLE1) GRID2 (gets info from TABLE2)
When you select an item in GRID2 it changes the BindID of GRID2 to filter the records down as the two tables are linked with a one to many relationship. When you click "Add" on GRID2 to add a record to "TABLE2", the form that displays has automatically established it's link to TABLE1, so any items from TABLE1 you have displaying on that form populate themselves immeditely (even in "Add Mode" - the form is just expecting you to fill out whatever values you want for TABLE2.
This is where is get's a bit whacky. I want to simulate the clicking of "Add" on GRID2 from elsewhere on the window rather than channeling the user into having to right-click on GRID2. Where I'm doing this from is irrelevant I guess, but for the sake of example it could be a button elsewhere on the form, or an additional menu item I have added to GRID1.
Do you know how I can do this, or am I a bit stuffed in this respect? As someone else has mentioned, many moons ago in legacy script you could call "DBGridAddItem" from the grid itself using script, and hey-presto it forced exactly the same reaction as selecting "Add" from the built in grid menu.
Thanks for your quick response though. What was it, about 2 minutes? Aren't you supposed to be at work? ;-) | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 2/24/2011 10:08:06 AM | fiogf49gjkf0d Typo... I don't think I can go back and edit my comment.
I meant...
"When you select an item in GRID*1* it changes the BindID of GRID2" | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 2/24/2011 10:11:19 AM | fiogf49gjkf0d Application.BasicFunctions.ShowAddForm
DOH!!!! | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 2/24/2011 11:00:44 AM | fiogf49gjkf0d Function ShowAddForm(ByVal TableName As String, ByVal ViewName As String, [ByVal BindDataPath], [ByVal BindValue]) As String
This appears that it would do the trick - unfortunately the SLX Dev Ref only mentions it in passing, and I haven't a clue what to pass for the BindDataPath. It looks like I'd have to pass something otherwise it won't tie the new item to the bound table. I've tried passing all but the BindDataPath and this doesn't work. I've also tried passing the table name, this doesn't work. I've also tried passing "TABLENAME:TABLEKEYFIELD" and this doesn't work.
Arrrrgh! | |
|
| Re: How to generically invoke the edit form for a SalesLogix datagrid Posted: 2/24/2011 11:25:02 AM | fiogf49gjkf0d Ok. Delete half of my ranting above if it's taking up unneccessary space. I've figured out how to use Application.BasicFunctions.ShowAddForm
Syntax: Function ShowAddForm(ByVal TableName As String, ByVal ViewName As String, [ByVal BindDataPath], [ByVal BindValue]) As String
To keep this simple. Let's say that there are two tables. ACCOUNT and ACCOUNT_BEAN. In ACCOUNT_BEAN we are storing all the different types of bean that the ACCOUNT in question enjoys. Baked, Kidney, Broad etc...
When I invoke ShowAddForm - I want to pass to it the relationship between the ACCOUNT_BEAN and the ACCOUNT itself to integrity is not lost.
For this, I have the following variables...
strACCOUNTID - The AccountID of the account the ACCOUNT_BEAN is related to strVIEWNAME - The name of the view I am invoking
I would then invoke the function like this...
Application.BasicFunctions.ShowAddForm "ACCOUNT_BEAN", strVIEWNAME, "ACCOUNT_BEAN:ACCOUNTID", strACCOUNTID
Really, really simple when you know how. Getting the knowhow is a different matter entirely! :-) | |
|
|
|
|
|
Visit the slxdeveloper.com Community Forums!
Not finding the information you need here? Try the forums! Get help from others in the community, share your expertise, get what you need from the slxdeveloper.com community. Go to the forums...
|
|
|
|
|
|