7/13/2025 3:29:31 AM
slxdeveloper.com
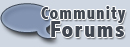 Now Live!
|
|
|
Dynamically Creating DataGrid Columns |
|
Description: |
In a previous article, I covered how to create datagrid columns at runtime. This article covers how to create DataGrid columns without any prior knowledge of the fields in the query. This article will assist you in creating dynamic DataGrids regardless of the fields in the query, or even the source of the query.
|
Category: |
SalesLogix ActiveX Controls
|
Author: |
Ryan Farley
|
Submitted: |
12/11/2002
|
|
|
Stats: |
Article has been read 77119 times
|
Rating:
    - 4.8 out of 5 by 13 users |
|
|
|
fiogf49gjkf0d
In a previous article, I covered how to create datagrid columns at runtime. This article covers how to create DataGrid columns without any prior knowledge of the fields in the query. This article will assist you in creating dynamic DataGrids regardless of the fields in the query, or even the source of the query.
In my previous article (see Creating DataGrid Columns at Runtime), I outlined how to create DataGrid columns at runtime. The problem with that article is that you had to hard code the columns you were setting up in the query. What if you wanted the query to be more dynamic? What if you wanted to build some unknown query based on conditions selected by the user? Wouldn't it be cool to have an interface where the user could select some values or check off some options and you would dynamically build the query and bind it to the datagrid? Sure it would. But that means that you would have to create the DataGrid columns without knowing what they are ahead of time.
The new DataGrid control in version 6 makes this easy. It exposes a crucial object to make this task (and many more) a breeze.
When you use the version 6 DataGrid, it basically takes the query passed to it in the DataGrid.SQL.Text property, creates a connection to the current SalesLogix database (or uses the connection string you pass to it in the ConnectionString property) and creates a Recordset to bind to. The great part about this is that it exposes the Recordset for you to get all kinds of useful information from it.
One of the great things you can get from the Recordset is some schema info about the fields it contains. The Recordset's Fields collection contains a lot of good information about the columns in your query as well as the data in each column. Each Field object in the Fields collection has a number of properties to provide you with schema information about that column. You can get the data type of a field, it's size, scale, precision, or any number of attributes like whether it accepts NULL values or not. For this article, we'll just look at one of these properties, the 'Name' property. You guessed it, this property gives us the name of the field in the query.
Note: If you'd like to see an article going into what is available in the Field object, let me know in the comments of this article and I'll put something together.
Ok, let's see how to do it. In the following code, we'll remove any existing columns in the DataGrid, set the query for it's SQL.Text property (we'll take the query from a Memo control named memSql), get a reference to the Recordset it is bound to and dynamically create the columns based on the fields in the query.
Dim rs
Dim col
Dim fld
Dim i
With DataGrid1
' Remove any existing columns
If (.Columns.Count > 0) Then
For i = 0 To .Columns.Count - 1
.Columns.Item(0).Delete
Next
End If
' Add new SQL and refresh so grid is bound
.SQL.Text = memSql.Text
.Refresh
' Pull the recordset from grid to determine fields in query
Set rs = .Recordset
For Each fld In rs.Fields
Set col = .Columns.Add(0)
col.FieldName = fld.Name
col.Caption = fld.Name
' If field ends in "ID" then assume that field is hidden
If Right(LCase(fld.Name), 2) = "id" Then col.Visible = False
Next
.ReadOnly = True
.RowSelect = True
.Refresh
End With
Not too bad, was it? I didn't think so. Collections are great to work with, I love 'em. But, before we look at what we got from the Fields collection, let's walk through what the code is actually doing.
First thing we did was to clear out any existing Columns we had in the DataGrid. This is the same as how we did this in my previous Creating DataGrid Columns at Runtime article. Nothing new. But to break out that part of the code, here it is:
If (DataGrid1.Columns.Count > 0) Then
For i = 0 To DataGrid1.Columns.Count - 1
DataGrid1.Columns.Item(0).Delete
Next
End If
Then we set the SQL for the DataGrid. In this example, I took the SQL from a Memo control named memSQL.
DataGrid1.SQL.Text = memSql.Text
DataGrid1.Refresh
Notice the 'Refresh' after setting the query. Why did we do this? Because, apparently, the DataGrid does not actually bind to the data in the query until a Refresh is called. We need the grid to be bound because we'll need to get a reference to the Recordset it is bound to. So we'll call Refresh here so the Recordset will be ready and waiting for us.
Now comes the fun part. We'll get a reference to the Recordset the DataGrid is bound to and the get the fields from it's fields collection.
Set rs = DataGrid1.Recordset
For Each fld In rs.Fields
Set col = DataGrid1.Columns.Add(0)
col.FieldName = fld.Name
col.Caption = fld.Name
' If field ends in "ID" then assume that field is hidden
If Right(LCase(fld.Name), 2) = "id" Then col.Visible = False
Next
We get the reference to the bound Recordset by setting a local Recordset object to the DataGrid.Recordset property. Then we enumerate the Fields in the Fields collection. With each of the Fields, we create a new DataGridColumn object (by calling the DataGrid.Columns.Add method and the DataGridColumn object is returned to us) and set the field it is bound to with the DataGridColumn.FieldName property. We set it's caption to the name of the Field name also via the DataGridColumn.Caption property. In this example, I am also setting any fields that end in "ID" as hidden fields in the DataGrid. Since the caption of the Columns in the DataGrid will be the field names, you can alias the field names in your query to make them display nice (ie: SELECT ACCOUNT AS Company, MAINPHONE AS Phone FROM ACCOUNT, etc).
Last thing we have to do is Refresh. The columns we have created will not appear until we Refresh again. I don't really like this because it means that we have to Refresh twice. Once to bind the data so we can get the fields from the bound Recordset and a second time to display our columns. However, this is not too major of an issue. Since SalesLogix is pooling the connections the hit is minor (because the connections are already opened, we're just opening the query).
Where could you take this? There are many additions you can add to this. For example, you could determine the data type of the underlying field and create the appropriate DataGridColumn type based on it's type. For example, creating Check columns for boolean/bit or char(1) fields and so on. You could wrap this up in an easy to use class to make it generic and reusable (want an article on this?). Gotta love that.
Until next time, happy coding.
-Ryan
|
|
|
|
Rate This Article
|
you must log-in to rate articles. [login here] 
|
|
|
Please log in to rate article. |
|
|
Comments & Discussion
|
you must log-in to add comments. [login here]
|
|
|
- subject is missing.
- comment text is missing.
|
|
| Question to the Author ;-) Posted: 12/11/2002 11:04:44 AM | fiogf49gjkf0d I see in your code your checking to see if the last two characters of the name are 'ID'. If this is true you hide the field. There are a lot of words out there that end in 'ID' is there a better way to do this. maby a nameing convention in the view names or other properties in the schema information? | |
|
| Re: Question to the Author... Posted: 12/11/2002 11:33:32 AM | fiogf49gjkf0d That is true, you wouldn't want to hide field names such as PUTRID, BICUSPID, or FLACCID (wow, wouldn't those be some useful fields!). Maybe a better approach would be to alias all fields with some standard convention to indicate it should be hidden. Then you could alias all fields you want hidden with something like MYFIELD AS NOVIS_MYFIELD and then look for any field starting with NOVIS and mark it as a hidden field. This way you could have extra details with each row in the grid to make it easy to grab when a user clicks on a row.
-Ryan
| |
|
| Neat Stuff Posted: 12/20/2002 8:42:11 AM | fiogf49gjkf0d Mike raises an interesting issue, and it ties in nicely where I'd like this to go.
We need a method on documenting the Data Field and Table usages in Sales Logix. One that is persistent despite Resetting Resynchtabledefs.....
We need a COMMENTS field, Key Views where the data is manually entered, major imports where the data could be changed, ownership rules etc. Plus the Display Name we already have.
Then we need to add the Query/Data Grid Default Attributes for each field......including Hidden.
If we have a Table SECTABLEDEFS_DOC or something like that would be fine. This Assumes that SLX will wipe out SECTABLEDEFS. SCocs would have all of the system documentation, and then the Data Grid defaults....then we set the Grid using these values.
The Default for all ----ID fields could be hidden....and then you could override......
In Ryan's case, the field to hide is ---ID where ---- = the name of the table. We don't want to hide SECODEID, ACCOUNTMANAGERID, USERID, COMPLETEDUSER kinds of fields in Account, Activity, History, et al. So if Default Format is ftNONE and the field ends in ID then we hide it...... but if the Default Format is ftUSER or ftOWNER and the field ends in ID then we don't hide it......
So.....
We need you to walk us through the next step: underlying data (META DATA) for an item....then populate the defaults in a permanent SLX table. Like a Resynch SecTableDefs routine that wouldn't wipe out existing records, but delete missing records (right join) and insert new records (left join) from this new table (no update).
I think SLX should be involved in this..... | |
|
| DataGrid Posted: 1/9/2003 11:12:47 AM | fiogf49gjkf0d Will there be more articles on using the DataGrid? I have been using the DataGrid for the last month. I have found some problems with the recordset object and also the ChangeNode Event. Would like to know if there is a fix for these problems or work arounds. Thanks | |
|
| Re: More articles on DataGrid... Posted: 1/10/2003 10:36:02 AM | fiogf49gjkf0d I do plan to write several more articles on the DataGrid. Some may get into how to work around some of the current problems & deficiencies the DataGrid has, but most will look at how to use more of the many undocumented parts of the DataGrid.
-Ryan
| |
|
| Question to All/Author Posted: 3/27/2003 10:04:12 AM | fiogf49gjkf0d Just wondering how to modify this so that we add conditions to the query w/o it trying to add the condition as a column. Trying to simplify the grid so it pulls info based on currentUserId.
strUserId = Application.BasicFunctions.CurrentUserID strSQL = "SELECT A1.LASTNAME, A1.FIRSTNAME, A1.ACCOUNT FROM CONTACT A1 LEFT JOIN ACTIVITY A2 ON (A1.CONTACTID=A2.CONTACTID) WHERE (A2.TYPE=262146) AND (A2.USERID= strUserId)"
and using the strSQL as the SQL.TEXT portion
Thanks for the help | |
|
| Re: Question to All/Author... Posted: 3/27/2003 10:51:43 PM | fiogf49gjkf0d As far as adding conditions, all you'd really need to do is add them to the where clause of the SQL string (and refresh if the gird was already set up).
Rich, in your example, all you'd need to change is to make sure you're appending the string containing the current userid. Like this:
strUserId = Application.BasicFunctions.CurrentUserID strSQL = "SELECT A1.LASTNAME, A1.FIRSTNAME, A1.ACCOUNT FROM CONTACT A1 LEFT JOIN ACTIVITY A2 ON (A1.CONTACTID=A2.CONTACTID) WHERE (A2.TYPE=262146) AND (A2.USERID= '" & strUserId & "')"
That's it. If the grid was already set up (ie: already has the columns) then you'll have to refresh it after adding the condition.
-Ryan | |
|
| Re: Question to the Author Posted: 10/24/2003 1:00:51 PM | fiogf49gjkf0d This may be a late entry but I think it might be useful.
In the resynctabledefs they have the key fields (id values) stored for every table. Since these are ONLY the id fields like accountid, custom_tableid, etc you could simply run a query to get the id fields from this table and check the entire recordset against these id fields to see if they match.
To look at the id fields for a table run the query: "select keyfield from resynctabledefs where tablename = table". If it's run on say the account table it produces a line with the accountid. The only problem here is if I run a query on a custom table with accountid and custom_tableid as the id fields only custom_tableid shows up in this query.
From there you could run another query: "select fromfield from joindata where fromtable = table" which would give you the account id field in your custom table if it's a one to many table. If it's a one to one relationship the fromfield here will equal the keyfield in resynctabledefs so you could add a check for this.
Anyways I believe through a join or a careful select statement one could produce both id fields at one time rather than perform 2 queries. This would be the most ideal but since they are select statements and you can read the returns and close the connections we aren't talking about a lot of used resources.
I could be wrong in my logic but this should work for now until SalesLogix changes the way it internally stores data. There's probably a bunch of other ways to do the same thing programmatically but I just happened to think of this one as the easiest in my head. If you have another method by all means share as I want to handle as much of this programmatically as possible. | |
|
| evaluating what the user selected from grid Posted: 10/29/2003 10:24:13 AM | fiogf49gjkf0d All,
I'm basically doing the same thing based on code I learned in the SLX developer class. The problem I'm having is I can't seem to get the value of the first field of the grid. Let’s say the user clicks on the second record in the grid and its highlighted. And behind the click event of a button I have dgEQP.GetCurrentField. This code is working correctly for a previous grid but for this grid the string come back empty. Any ideas? | |
|
| Re: evaluating what the user selected from grid... Posted: 11/11/2003 12:24:39 AM | fiogf49gjkf0d What happens if you reference the field by name (instead of grabbing the key field value by not specifiying a name)?
ie: val = dgEQP.GetCurrentField("myfieldname")
Also, do you have the grid grouped by a column? I know that this can at times effect what GetCurrentField returns.
-Ryan
| |
|
| Setting Column Width Posted: 3/25/2004 9:41:54 PM | fiogf49gjkf0d This is great! I created a Lookup View for every key field in our database just by adding couple extra lines to your code. Is it possible to set the column width, say I want 1st column to be 100 px and second column to be 30 px etc. ? Also where do you find references for the fields collection etc ? | |
|
| Re: Dynamically Creating DataGrid Columns Posted: 3/25/2004 10:49:38 PM | fiogf49gjkf0d Binu, to set the width you could just follow this up with lines such as:
With datagrid1.Columns .Item(0).Width = 150 .Item(1).Width = 75 End With
As far as the Fields collection, that is part of ADO. If you google for "ADO Fields collection" I am sure you'll find many resources. Also there is a good ADO reference book featured on the home page of this site.
-Ryan
| |
|
| Re: Dynamically Creating DataGrid Columns Posted: 3/26/2004 3:57:07 PM | fiogf49gjkf0d First I will like to thank you for all the precious help that you share with us.
Now about your creating dynamic grid code, I put it in my functions library and right now I call it with a function FillMyGrid(StrSQL) but I will like to supply my SQL query and the name of the grid in my call but right now I have a error it seem that it doesn’t like my GridName variable (FillMyGrid(StrSQL,StrMyGridName). Can you give me any suggestions?
Thank-you and have a nice day
| |
|
| Re: Dynamically Creating DataGrid Columns Posted: 3/26/2004 4:06:34 PM | fiogf49gjkf0d Christian,
You *don't* want to pass the grid name as a string. Instead you pass it as an object. I have a script where I do the same. I pass the grid itself and the SQL string.
For example, if I have a grid on a form named "datagrid1", and I want to pass it to a generic FillData method, I would pass it like this:
FillData datagrid1, "select * from account"
And the FillData method would look something like this:
Sub FillData(grid, sql)
With grid ' now do the stuff with the grid object ' ... .SQL.Text = sql ' ... End With End Sub
Got it? -Ryan | |
|
| Re: Dynamically Creating DataGrid Columns Posted: 3/27/2004 6:29:11 AM | fiogf49gjkf0d Got It !! and it's working like a charm thank ;-) | |
|
| Re: Dynamically Creating DataGrid Columns Posted: 2/3/2005 6:38:42 AM | fiogf49gjkf0d How does one format the column type during runtime, for eg i would like to change the format type from standard to combo type property. I dont see anything in the documentation. Can i do something like this
datagrid1.columns(i).type="Combo" or is there a Long Integer Value that i need to specify instead of a text string like "Combo".
Kannan Srinivasan | |
|
| Re: Dynamically Creating DataGrid Columns Posted: 2/3/2005 11:25:52 AM | fiogf49gjkf0d Hi Kannan,
To use a different column type, other than standard, you would create it as that type. This would create a Combo column:
Set col = DataGrid1.Columns.Add(8)
I listed the column types for version 6.0 in a previous article: http://www.slxdeveloper.com/devhome/page.aspx?id=35&articleid=6 However, one thing to know, working with specific column types only works in 6.2. The needed interfaces & objects were not exposed in v6.0-v.6.1.
I'll actually have an article at some point on working with these in v6.2, but that all depends on my work load to get that done, too busy at the moment ;-)
-Ryan | |
|
| Re: Dynamically Creating DataGrid Columns Posted: 2/17/2005 10:19:45 AM | fiogf49gjkf0d This is probably simple but does anyone know how to make the columns sortable after they are created dynamically? I tried to add col.sorted = 1 under each Set col = .Columns.Add(0) but that didn't work. Also I tried to add .Sortable = True at the end of the code to specify the datagrid properties but that doesn't work either. Any help would be appreciated because I am stuck.
Thanks for the help. | |
|
| Re: Dynamically Creating DataGrid Columns Posted: 3/15/2005 6:47:16 AM | fiogf49gjkf0d It seems to me that there is a upper boundary for the .sql.text.
im trying to parse a querystring with the length of 1600+ characters, and it seems to fail.
is this right??? | |
|
| Re: Dynamically Creating DataGrid Columns Posted: 3/15/2005 7:49:30 AM | fiogf49gjkf0d Christian,
There's no string length limit that I've ever run into. The problem is likely nothing to do with the length, but moreso with the syntax of the query. Keep in mind that the provider does pick apart every SQL statement and rebuilds it so that I can append the appropriate security details into the query. If you're using some combination of syntax that the provider has a hard time understanding then it will fail, even though it might be a valid SQL statement when running it in Query Analyzer. If you're using 6.2 you can use SQL views, maybe that is a route you could take? Otherwise it is a matter of reconstructing the query yourself to find some syntax that the provider does not have issues with.
-Ryan | |
|
| DateTime Column Style in a Data Grid Posted: 3/28/2005 5:30:05 PM | fiogf49gjkf0d I am Dynamically creating the Datagrid but one of the columns I need to have a DateTime field. Which I set the Style type to DateTime I get a " is not a valid floating point value." error message. Did I miss something when set up the column? Here is a snippet: Set col = grdProducts.Columns.Add(3) col.FieldName = "START_DATE" col.Caption = "Start Date" col.FormatType = ftDataTime col.Width = 65
Ed-New SLX Developer | |
|
| Jump to Value in DataGrid ? Posted: 4/18/2005 10:52:39 PM | fiogf49gjkf0d In 5.2 I was using an EditBox to 'QueryJumpToTypeAhead' where the user enters text into the EditBox and the DataGrid dynamically 'jumps' to the value/s the user is typing.
How is this done in 6.2 VB Script ? | |
|
| Re: Dynamically Creating DataGrid Columns Posted: 12/28/2005 2:51:32 PM | fiogf49gjkf0d I like how you explained this article versus of reading my book.
How would you get a specific data that are in different col into grid that is in the form? | |
|
| Re: Dynamically Creating DataGrid Columns Posted: 12/28/2005 2:56:58 PM | fiogf49gjkf0d Hi Mary,
I'm not sure I understand your question, could you restate?
Thanks, Ryan | |
|
| Re: Dynamically Creating DataGrid Columns Posted: 12/28/2005 8:45:45 PM | fiogf49gjkf0d First I want to THANK YOU for responding so fast. Please excuse me if I am not clear. I am just learning the VB6 coding and SQL.
I'm doing a project where I would use the acct# to retrieve some infomation in the database. I need 5 piece of info of the file and I would like to have that 5 specific piece of info in my grid on the form that matches the header. I got the connection right but the information I want is not under the header.
Thank you, | |
|
| Re: Dynamically Creating DataGrid Columns Posted: 1/23/2006 5:04:04 PM | fiogf49gjkf0d Has there been any resolution to the Sortable issue with Dynamic Grids? I can not set them to sortable | |
|
| Re: Dynamically Creating DataGrid Columns Posted: 2/6/2006 9:42:54 AM | fiogf49gjkf0d Billy,
As far as sortable grids go, what I usually do is create the grid at design time and then set things to sortable, but then at run time I just do my own stuff. IIRC the sortable setting usually sticks in this case.
-Ryan | |
|
| Re: Dynamically Creating DataGrid Columns Posted: 2/7/2006 12:10:41 PM | fiogf49gjkf0d If I set the Grid to Sortable it will only Show Data if the Columns Match the Default Columns that are created when the datagrid is created. Otherwise nothing gets displayed. | |
|
| Re: Dynamically Creating DataGrid Columns Posted: 11/6/2006 3:06:54 PM | fiogf49gjkf0d I have created a grid dynamically. All data fills in correctly and row select is set to true.
When a row is selected I cannot get the RowSelect event to fire.
What am I missing ?
| |
|
| Re: Dynamically Creating DataGrid Columns Posted: 6/20/2007 8:16:20 PM | fiogf49gjkf0d Im trying to retrieve record during runtime using datagrid. Im getting a compile error method or data member not found.....How will I solve this problem? Am I missing something?Do I need to download the SLXSaleslogic.ocx? Thanks | |
|
| Re: Dynamically Creating DataGrid Columns Posted: 6/20/2007 10:35:39 PM | fiogf49gjkf0d Ferdi,
What does your code look like now? Are you trying to get the "Selected" row only, or any particular row? Does your grid have MultiSelect set to True or False? What version of SLX are you on?
-Ryan | |
|
| Re: Dynamically Creating DataGrid Columns Posted: 6/21/2007 12:05:09 AM | fiogf49gjkf0d Hi Ryan,
My code is..... Im using the default datagrid of VB6.... Do I need to use the SLX ocx?where can I download it? Thanks
Dim col Dim i
With DataGrid1 .SQL.Text = "SELECT header_id, order_no, ordered_date FROM so_header"
With .Columns 'remove any existing columns If (.Count > 0) Then For i = 0 To .Count - 1 .Item(0).Delete Next End If
'add column for header_id field Set col = .Add(0) col.FieldName = "header_id" col.Caption = "Header ID" col.ReadOnly = True
'add column for order_no field Set col = .Add(0) col.FieldName = "order_no" col.Caption = "Order No" col.ReadOnly = True 'add column for ordered_date field Set col = .Add(0) col.FieldName = "ordered_date" col.Caption = "Order Date" col.ReadOnly = True
End With 'now refresh the grid to see the new columns .Refresh End With | |
|
| Re: Dynamically Creating DataGrid Columns Posted: 1/10/2008 8:21:21 PM | fiogf49gjkf0d Hi Guys,
I am not able to edit the datagrid means it always readonly. Please find my code below which i am trying. I am working on the version 6.2.6.1007. Guys please advise why i am not able to edit the grid is there any other way which i can use to edit the grid.
Details: Bind id is bound to contactid. and key filed is contactid also for the grid.
Sub BuildDataGrid Dim col,i Dim strSQL Dim rs Dim fld strSQL = "SELECT PAM.PROCESSAUDITMASTERID,CP.ISSELECTED,PAM.STAGENAME + ' - ' + CONVERT(VARCHAR,PAM.PROBABILITY,20) + '%' STAGE,PAM.STEPNAME,CP.DESCRIPTION," _ & "CP.STARTEDON,CP.COMPLETEDON FROM sysdba.PROCESSAUDITMASTER PAM LEFT JOIN sysdba.CONTACTPROCESS CP ON " _ & "(PAM.PROCESSAUDITMASTERID = CP.PROCESSMASTERAUDITID AND CP.CONTACTID = " & "'" & Application.BasicFunctions.CurrentContactID & "'" & ")"
with grdCampaign If (.Columns.Count > 0) Then For i = 0 To .Columns.Count - 1 .Columns.Item(0).Delete Next End If .SQL.Text = strSQL .Refresh .ReadOnly = False Set rs = .Recordset For Each fld In rs.Fields if fld.Name = "ISSELECTED" then Set col = .Columns.Add(4) col.Valuechecked = True col.valueunchecked = False col.Caption = Application.Translator.Localize("Select") col.width= 50 ELSEIF fld.Name = "PROCESSAUDITMASTERID" then SET col = .Columns.Add(0) col.Caption = fld.Name col.FieldName = fld.Name col.width= 10 col.Visible = false ELSEIF fld.Name = "STAGE" then SET col = .Columns.Add(0) col.Caption = fld.Name col.FieldName = fld.Name col.width= 180 ELSEIF fld.Name = "STARTEDON" OR fld.Name = "COMPLETEDON" then SET col = .Columns.Add(3) col.Caption = fld.Name col.FieldName = fld.Name col.width= 150 ELSE SET col = .Columns.Add(0) col.Caption = fld.Name col.FieldName = fld.Name col.width= 300 END IF Next .ReadOnly = False .Refresh end with End Sub | |
|
| Dynamically Creating DataGrid Columns Posted: 2/10/2008 2:30:50 AM | fiogf49gjkf0d Hi Ryan, I'm using SLX 7.0 and I have created a custom table that stores all the customer transactions and depending on the client it will list down the transactions in a datagrid. I did it without coding in Architect in a simple way, which is working fine and get few minutes to load when there are 1000 records.. but need to know how to do paging if I need to restrict the datagrid to show only 20 rows at time and next button and previous button handle the rest to navigate the information.
SELECT A1.WS_TRANSACTIONID, A1.CRTRNPCD, A1.CRTRNSPC, A1.CRTRNRNO, A1.CRTRNBKD, A1.CRTRNDOT, A1.CRTRNTOT, A1.CRTRNPCAM, A1.CRTRNCCRG, A1.CRTRNRAM, A1.CRTRNTCAM, A1.CRTRNCERT, A1.CRTRNTRNS, A1.CRTRNBNM, A1.CRTRNTTYP FROM WS_TRANSACTION A1 WHERE A1.CONTACTID = :BindID
this is the SQL generated by the SLX, but can I add one more condition to the A1.WS_TRANSACTIONID and pass the value to the SQL query.. to make ease the coding work without altering the current context..
Hope you can help on this to me!!!! | |
|
|
|
|
|
Visit the slxdeveloper.com Community Forums!
Not finding the information you need here? Try the forums! Get help from others in the community, share your expertise, get what you need from the slxdeveloper.com community. Go to the forums...
|
|
|
|
|
|